Improving Classroom Engagement with ChatGPT
A step-by-step guide to creating and using a custom AI tool powered by Google Forms and OpenAI APIs for gathering and analyzing student opinions
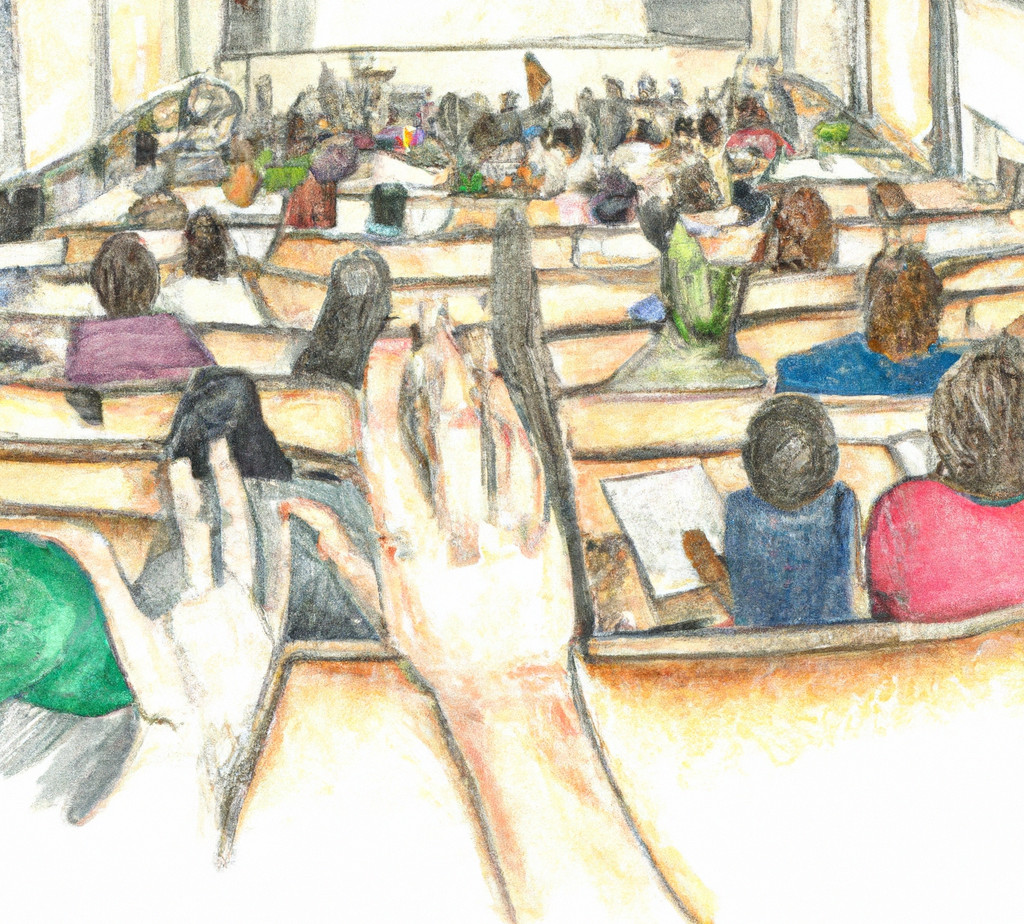
As a Law Professor (@University of Milan), I am constantly looking for ways to improve the learning experience for my students.
One aspect that I find particularly important is participation in class. Active participation not only helps students learn better but also creates a more engaging and dynamic classroom environment.
However, in large classes, it can be challenging to listen to, and take into account, the opinion of each individual student. With limited class time, it is simply not feasible to hear from everyone.
To solve this problem, I developed a software tool that gathers the opinions of all students in the class and summarizes them in bullet points using OpenAI API's. The tool uses a Google Form to gather responses from students, which are then analyzed by OpenAI's natural language processing capabilities to identify common themes and summarize the responses.
Once the responses have been summarized, they are automatically inserted into a slide, which I can then use to analyze the responses and facilitate discussion with the class. This tool has been a game-changer for me in terms of gathering and analyzing student feedback in large classes.
In this post, I will share the logic and step-by-step process behind creating this software. Additionally, I will provide the code necessary for anyone to replicate this system in their own classroom. With this tool, every educator can efficiently gather and analyze students' feedback, regardless of class size, their budget or technical expertise.
Please note that all licensing information for the software and the code used in this post is provided at the end of the post.
My Real World Experience Using this Tool
I have already used this tool in one of my classes, and the response from students was overwhelmingly positive.
When asked the question What does sustainable development mean for you? students expressed a variety of responses, including:
- The process our society (individuals, companies, P.A.) should follow
- The effort to create a world that the future generations can live in good conditions, i.e. not giving up on qualities of life which we have or older generations might have had during their lifespan
- The key to change the world in order to assure human well-being
- The competitive environnent, the focus on profit and the attitudes need to change
- Development that satisfies the needs of the present generation and provide the same or better for the future
- placing the environment and a bettering of social conditions at the heart of public policy
- As a citizen of a country that has abundant natural resources, sustainable development means using these resources in a responsible and efficient way to benefit the present and future generations.
- Outcome of compliance with environmental rules elaborated by specialists
- To invest in a better way of dealing with the world so that it remains better in the future
- Reaching better living standards for every member of society, ensuring progress, welfare and human and natural protection in a way that will be enjoyed by future generations too
- Careful and thought out use of resources
- It means living in a way that pollute less. Empower policies that can help the environment and the ‘common goods’ to be saved. Try to reduce useless stuff that can pollute more.
- It is a current development without limiting future generations to satisfy their needs in the future.
- I believe it has to be seen from different points of views, as an environmental issue and also economical, meaning how can we achieve economic development sustainably, for everyone
- To preserve not only the environment but also the other dimensions (society and economy) and save them for the future generations.
- It means our society can continue its way step by step
- Sustainable development means cooperate and finding ways to live in our current developed world without damaging even more the natural resources we still have
- Outcome of compliance with environmental rules elaborated by specialists
- Trying to take into consideration the environmental, social and economic aspect in everything we do without compromising future generations' needs
- Using Renewable Energy which will eco friendly.
- Sustainable development is the development which coincides with the present goals without posing a threat for the future of all society members
- The development of public policies in collaboration with all the affected stakeholders and the effective enforcement of it in balance between economic, social and environmental aspects
- Economic development by using renewable resources
- Sustainable development means implementation of steps for unstoppable growth in various spheres of life (of humans: on social, individual level), transformations into new levels of condition
- Achieving a system that is consistent and equal for every individual
- A development that can be defined as sustainable is that process of amelioration of economics, society and legislative sphere with the purpose of reconcile the relationship between man and nature
Using this tool, I was able to summarize these responses as follows:
- Society should aim to create a world that future generations can thrive in
- The key to achieving human well-being is changing attitudes towards competition and profit
- Development should meet the needs of the present while providing for the future
- The environment and social conditions should be at the heart of public policy
- Sustainable development means using resources responsibly to benefit present and future generations
- Compliance with environmental rules is important for outcomes
- We must invest in a better future for the environment and living standards for all members of society
- Sustainable development involves careful and thoughtful use of resources
- It's about preserving not only the environment but also society and the economy
- Sustainable development involves using renewable resources and implementing steps for growth in all spheres of life
The class was able to have a meaningful and productive discussion on the topic, and I was able to use the summarized responses to guide the conversation and ensure that every student's opinion was taken into account.
In short, the tool proved to be an effective way to encourage participation and facilitate discussion.
Core Logic: How the Software Gathers and Analyzes Student Opinions
To implement the software, I decided to use a combination of Google Forms and Google Slides, as they are free and easy to use for both students and educators.
Firstly, I created a Google Form to gather opinions from the students during class. To direct students to the form, I generated a QR code of the form's link and inserted the image in the slides. During class, the slides are projected and students can easily access the Google Form with their smartphones.
Next, the opinions gathered via the Google Form are processed by the AI model gpt-3.5-turbo
by OpenAI and then the processed result is automatically inserted into a Google Presentation slide. To achieve this, the software uses the Google Presentation id
to identify the specific presentation and the Google Form id
to determine the slide in which to insert the responses.
To make the code as simple and reusable as possible, I have designed it to interact with each component with minimal configuration. By using the id
of both the Google Presentation and the Google Form, the software can seamlessly integrate the two and automate the process of gathering and analyzing student feedback.
In the next step-by-step guide, I will assume that you know how to obtain the id
of a Google Presentation and a Google Form. If you are unfamiliar with this process, a quick Google search will provide you with easy-to-follow instructions.
Step-by-Step Guide
How to Create the Software
Here is the step-by-step guide to implementing the software:
Go to App Script and create a new project. Let's call it
FormsResponsesToBulletPoints
.On the left side of the screen, you will see a list of files. One of these files will end in
.gs
(the name may differ based on your language). Rename this file toindex.gs
.
Copy and paste the following code into index.gs
:
function generateSummary(formId, apiKey) {
var metadata = getFileDescription(formId)
var responses = getFormResponses(formId)
var NUMBER_OF_EXPECTED_RESPONSES = 20
if((!responses || responses.length < NUMBER_OF_EXPECTED_RESPONSES) && !responses?.some(text => text?.toLowerCase()?.includes('xtrigger'))) {
return
}
// responses = responses.filter(text => !text?.toLowerCase()?.includes('xtrigger'))
var inputText = `Summarize and rephrase the following text in less than 11 bullet points. Return the result as a JSON formatted array of strings containing only the responses.\n\n ${responses.join(' ')}`;
var arrayResponses = fetchFromOpenAI(inputText, apiKey);
insertResultsIntoSlide(arrayResponses, metadata.presentationId, formId)
}
The above code includes a feature to limit the costs of using OpenAI's API by processing the responses only when the number of expected responses is more than a certain amount. The number of expected responses is defined by the
NUMBER_OF_EXPECTED_RESPONSES
variable, which is set to 20 in the code snippet. TheNUMBER_OF_EXPECTED_RESPONSES
variable can be edited to a value that suits your needs. This allows you to tailor the software to your specific class size, and budget.The code also includes the option to manually trigger the processing of the responses by submitting the phrase "xtrigger" (case insensitive) as a response. If any response includes this phrase, the system will process the responses regardless of the number of expected responses.
Now we need to add the rest of the logic.
- In the files list header, click on the ➕ icon to create a new file.
- Name the file
openai-helper
Copy and paste the following code into it:
function fetchFromOpenAI (inputText, apiKey) {
var openaiUrl = 'https://api.openai.com/v1/chat/completions';
var headers = {
'Content-Type': 'application/json',
'Authorization': 'Bearer ' + apiKey
};
data = {
model: 'gpt-3.5-turbo',
messages: [
{
content: inputText,
role: 'user'
}
]
};
var options = {
'method': 'post',
'headers': headers,
'payload': JSON.stringify(data)
};
var response = UrlFetchApp.fetch(openaiUrl, options);
var summaryText = JSON.parse(response).choices?.[0]?.message?.content
var arrayResponses = []
try {
arrayResponses = JSON.parse(summaryText.trim())
} catch (error) {
console.error(error);
}
return arrayResponses
}
- In the files list header, click again on the ➕ icon to create a new file.
- Name the file
get-forms-responses
Copy and paste the following code into it:
function getFormResponses(formId) {
var form = FormApp.openById(formId)
var formResponse = form.getResponses();
// Store the responses in an array
var responses = [];
formResponse.forEach(function(response) {
var itemResponses = response.getItemResponses();
var responseArray = [];
itemResponses.forEach(function(itemResponse) {
responseArray.push(itemResponse.getResponse());
});
responses.push(responseArray);
});
responses = responses.flat()
return responses
}
- In the files list header, click again on the ➕ icon to create a new file.
- Name the file
insert-result-into-slide
Copy and paste the following code into it:
function insertResultsIntoSlide(arrayResponses, presentationId, formId) {
var presentation = SlidesApp.openById(presentationId);
var slides = presentation.getSlides()
var slideIndex = slides.findIndex(slide => {
var notesPage = slide.getNotesPage()
var speakerNotes = notesPage?.getSpeakerNotesShape()
var speakerNotesShape = speakerNotes?.getText()
return speakerNotesShape?.asString()?.includes(formId)
})
var summaryText = arrayResponses.join('\n\n') + 'AI tool developed by Gherardo Carullo'
// Insert the summary text into a Google Slides presentation
var slide = slides[slideIndex];
var shape = slide.getShapes()[1];
shape.getText().setText(summaryText);
}
- In the files list header, click once more on the ➕ icon to create a new file.
- Name the file
drive-file-info
Copy and paste the following code into it:
function getFileDescription(formId) {
var formFile = DriveApp.getFileById(formId);
return JSON.parse(formFile.getDescription()?.trim());
}
We are done creating the core of the software, the next step is to implement it with a specific presentation and form.
We will need to reference the id
of the script project we just created. To do so, on the left click on icon ⚙ (Project Settings) and copy the id
of the stript (it is a series of letters and numbers, something like: rgtmRp0xrA3wqhe2t…6713ZNtzwzj4Xnr14). Keep this for future reference.
How to Use the software
Using the software is straightforward and can be done in a few simple steps. Here is a detailed guide:
- Create a Google Presentation and a Google Form to collect student responses. Save the
id
s of both somewhere for future reference. - Make sure the form has only one question and answer. The software is designed to insert the answer into one slide, so using one question per form will ensure that the answer is inserted into one slide as expected.
- Open Google Drive and go to the folder where the form is located. Click on the form without opening it, and the details panel should appear on the right. Alternatively, right-click on the form and select "Details" from the menu.
In the description field at the end of the details panel, enter the following text:
{
"presentationId": "YOUR_PRESENTATION_ID"
}
Replace
YOUR_PRESENTATION_ID
with the appropriateid
of the presentation. Make sure to surround the value with double quotes"
. This step will allow the software to identify the correct presentation to insert the responses into.
- Optional: add a slide with the QR code and URL to the form so that students can easily access it and submit their responses. A good online tool to generate QR codes is qr-code-generator.com.
- Open the Google Presentation and go to the slide where you want to enter the responses. Ensure that this slide has exactly two shapes: one for the title and one for the contents of the slide. Most slide templates already have this format, so you may not need to make any changes.
- In the presenter notes section of the slide (located at the bottom of the slide edit view), enter the Form
id
. You can also add additional text, but make sure to include the Formid
. Thisid
is needed to identify the slide where the responses will be inserted. - Now open the Google Form and click on three dots menu located in the top right corner, and then click on the Script Editor option.
- On the left there is a Library section. Click on the ➕ icon and enter the
id
of the script you copied earlier. Click on the Search button and then on the Add button to add the script to the project.
Now copy and paste the following code in the code editor:
var formId = "YOUR_FORM_ID"
var apiKey = "YOUR_OPENAI_API_KEY";
function start() {
FormsResponsesToBulletPoints.generateSummary(formId, apiKey)
}
Replace
YOUR_FORM_ID
with the appropriate ID of the form. Make sure to surround the value with double quotes"
. This step allows the software to identify the correct form to collect the responses from.Replace
YOUR_OPENAI_API_KEY
with the appropriate API key. Make sure to surround the value with double quotes"
. To get an API key, you need to create an account on OpenAI. You can do so by clicking here.
We are almost done! The last step is to set a trigger to run the script. Please note that in this step Google will ask you to authorize the script to access your Google Drive and Google Slides. You can proceed without worrying about your data, as the script will execute entirely on your own Google account and the only data that will be shared with OpenAI is the text of the responses.
To do so, click on the clock icon on the left menu bar (Current project's triggers option). Click on the Add trigger button. You can leave the default settings, except for the following:
Type of event
: select to run the script only once the form is submitted (the exact phrasing may vary depending on your language settings).
Click on the Save button and you are done!
Conclusion
This software tool has proven to be a valuable asset in my classroom, allowing me to efficiently gather and analyze student feedback even in large classes.
By sharing the logic and step-by-step process behind creating this software, I hope to make it accessible to educators around the world who are looking for ways to improve their students' learning experience. With the code provided, anyone can easily replicate this system and integrate it into their own classroom.
This tool not only simplifies the process of gathering feedback but also enables educators to create a more inclusive and collaborative learning environment. I encourage all educators to give it a try and see the benefits for themselves.
License
I'm releasing this software and the code used in this post as open source, meaning that anyone can use them freely. However, I kindly request that you maintain attribution by including the statement "AI tool developed by Gherardo Carullo" in the final output slide of your presentation. This attribution statement can either be left as an output of the provided code, or added manually to the slide in a visible location.